Create a LINQ to SQL
continue and make the connection drop the table to DataClasses1.dbml
Method 1 (PHP like)
1-I write this code for WebForm1.aspx :
so now when I run the PRJ I have
2-is time to save the form data..
in form we have
the WebForm1_save.aspx through LINQ
the WebForm1_save.aspx through SQL
warning the 'HTML input controls' didnt converted to 'ASP.NET controls' (aka to be available at codebehind). The trick is to use Request.Form[elementname].
Method 2 (ASPX like) with UpdatePanel1/ScriptManager1 without ASPX.CS
just the button event merge on top on page..
when setting the ChildrenAsTriggers to false, asp.net stops to clear the input controls on save (great) BUT doesnt show us the red label...
continue reading at https://kedarnadhsharma.wordpress.com/2013/09/13/childrenastriggers-in-updatepanel/
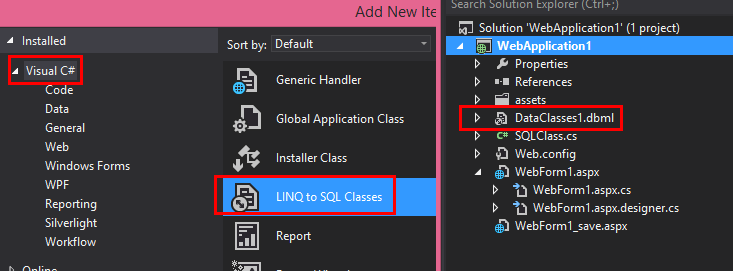
continue and make the connection drop the table to DataClasses1.dbml
Method 1 (PHP like)
1-I write this code for WebForm1.aspx :
JavaScript:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="WebForm1.aspx.cs" Inherits="WebApplication1.WebForm1" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" />
<script type='text/javascript' src='assets/jquery-1.11.0.min.js']</script>
<script src="assets/bootstrap.min.js"></script>
<link href="assets/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-4">
</div>
<div class="col-md-4">
<form id="form1" method="post" action="WebForm1_save.aspx">
<% WebApplication1.DataClasses1DataContext db = new WebApplication1.DataClasses1DataContext();
IEnumerable<WebApplication1.customer> customers = from p in db.customers
select p;
%>
<div class="form-group">
<label>Name :</label>
<input id="cust_name" name="cust_name" type="text" maxlength="50" class="form-control" />
</div>
<div class="form-group">
<label>Address :</label>
<input id="cust_address" name="cust_address" type="text" maxlength="50" class="form-control" />
</div>
<div class="form-group">
<label>PO Box :</label>
<input id="cust_po" name="cust_po" type="text" maxlength="10" class="form-control" />
</div>
<div class="form-group">
<label>Site :</label>
<select id="site_id" name="site_id" class="form-control">
<%
foreach (var item in customers)
{%>
<option value="<%= item.cust_id %>"><%= item.cust_name %></option>
<%} %>
</select>
</div>
<button class="btn btn-default" type="submit">Save</button>
</form>
</div>
<div class="col-md-4">
</div>
</div> <%--row--%>
</div> <%--container--%>
</body>
</html>
so now when I run the PRJ I have
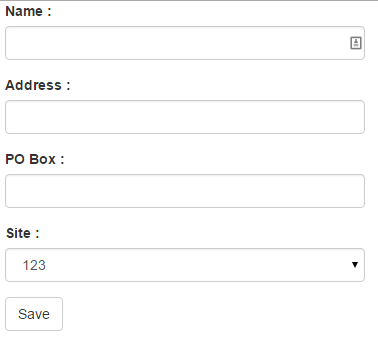
2-is time to save the form data..
in form we have
JavaScript:
<form id="form1" method="post" action="WebForm1_save.aspx">
the WebForm1_save.aspx through LINQ
JavaScript:
<%@ Page Language="C#" %>
<%
//Request.Form is filled only when something POSTED to page
if (Request.Form["cust_name"] == null || Request.Form["cust_address"] == null || Request.Form["cust_po"] == null || Request.Form["site_id"] == null)
{
Response.Write("Please fill the textboxes");
}
else
{
if (String.IsNullOrEmpty(Request.Form.Get("cust_name")))
{
Response.Write("cust_name is required");
return;
}
}
WebApplication1.DataClasses1DataContext db = new WebApplication1.DataClasses1DataContext();
WebApplication1.customer customer = new WebApplication1.customer
{
cust_name = Request.Form["cust_name"],
cust_address = Request.Form["cust_address"],
cust_po = Request.Form["cust_po"],
site_id = int.Parse(Request.Form["site_id"])
};
db.customers.InsertOnSubmit(customer);
bool status = false;
try
{
db.SubmitChanges();
status = true;
}
catch (Exception e)
{
Console.WriteLine(e);
}
if (status)
Response.Redirect("WebForm1.aspx");
else
Response.Write("save error!");
%>
the WebForm1_save.aspx through SQL
JavaScript:
<%@ Page Language="C#" %>
<%
//Request.Form is filled only when something POSTED to page
if (Request.Form["cust_name"] == null || Request.Form["cust_address"] == null || Request.Form["cust_po"] == null || Request.Form["site_id"] == null)
{
Response.Write("Please fill the textboxes");
}
else
{
if (String.IsNullOrEmpty(Request.Form.Get("cust_name")))
{
Response.Write("cust_name is required");
return;
}
}
System.Data.SqlClient.SqlConnection db = new System.Data.SqlClient.SqlConnection("Data Source=x;Initial Catalog=x;User ID=x;Password=x");
db.Open();
if (db == null || db.State!=System.Data.ConnectionState.Open)
{
Response.Write("**CONNECTION ERROR**");
return;
}
string sql = "insert into bbbcustomers values (@cust_name, @cust_address, @cust_po, @site_id)";
var sqlco = new System.Data.SqlClient.SqlCommand(sql, db);
sqlco.Parameters.AddWithValue("@cust_name", Request.Form["cust_name"]);
sqlco.Parameters.AddWithValue("@cust_address", Request.Form["cust_address"]);
sqlco.Parameters.AddWithValue("@cust_po", Request.Form["cust_po"]);
sqlco.Parameters.AddWithValue("@site_id", Request.Form["site_id"]);
int status = sqlco.ExecuteNonQuery();
if (status==1)
Response.Redirect("WebForm1.aspx");
else
Response.Write("save error!");
%>
warning the 'HTML input controls' didnt converted to 'ASP.NET controls' (aka to be available at codebehind). The trick is to use Request.Form[elementname].
Method 2 (ASPX like) with UpdatePanel1/ScriptManager1 without ASPX.CS
just the button event merge on top on page..
JavaScript:
<%@ Page Language="C#" %>
<%@ Import Namespace="System.Drawing" %>
<!DOCTYPE html>
<script runat="server">
protected void Unnamed1_Click(object sender, EventArgs e)
{
WebApplication1.DataClasses1DataContext db = new WebApplication1.DataClasses1DataContext();
WebApplication1.customer customer = new WebApplication1.customer
{
cust_name = Request.Form["cust_name"],
cust_address = Request.Form["cust_address"],
cust_po = Request.Form["cust_po"],
site_id = int.Parse(Request.Form["site_id"])
};
db.customers.InsertOnSubmit(customer);
bool status = false;
try
{
db.SubmitChanges();
status = true;
}
catch (Exception ex)
{
Console.WriteLine(ex);
}
if (status)
{
lbl_status.Text = "saved";
lbl_status.ForeColor = Color.Red;
}
else
Response.Write("save error!");
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" />
<script type='text/javascript' src='assets/jquery-1.11.0.min.js']</script>
<script src="assets/bootstrap.min.js"></script>
<link href="assets/bootstrap.min.css" rel="stylesheet">
<title></title>
</head>
<body>
<form id="form2" runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server">
</asp:ScriptManager>
<asp:UpdatePanel ID="UpdatePanel1" UpdateMode="Conditional" runat="server">
<ContentTemplate>
<div class="container">
<div class="row">
<div class="col-md-4">
</div>
<div class="col-md-4">
<% WebApplication1.DataClasses1DataContext db = new WebApplication1.DataClasses1DataContext();
IEnumerable<WebApplication1.customer> customers = from p in db.customers
select p;
%>
<div class="form-group">
<label>Name :</label>
<input id="cust_name" name="cust_name" type="text" maxlength="50" class="form-control" />
</div>
<div class="form-group">
<label>Address :</label>
<input id="cust_address" name="cust_address" type="text" maxlength="50" class="form-control" />
</div>
<div class="form-group">
<label>PO Box :</label>
<input id="cust_po" name="cust_po" type="text" maxlength="10" class="form-control" />
</div>
<div class="form-group">
<label>Site :</label>
<select id="site_id" name="site_id" class="form-control">
<%
foreach (var item in customers)
{%>
<option value="<%= item.cust_id %>"><%= item.cust_name %></option>
<%} %>
</select>
</div>
<asp:Button class="btn btn-default" Text="Save" runat="server" OnClick="Unnamed1_Click" />
</div>
<div class="col-md-4">
<asp:Label runat="server" ID="lbl_status"/>
</div>
</div>
<%--row--%>
</div>
<%--container--%>
</ContentTemplate>
</asp:UpdatePanel>
</form>
</body>
</html>
when setting the ChildrenAsTriggers to false, asp.net stops to clear the input controls on save (great) BUT doesnt show us the red label...
JavaScript:
<asp:UpdatePanel ID="UpdatePanel1" UpdateMode="Conditional" ChildrenAsTriggers="false" runat="server">
continue reading at https://kedarnadhsharma.wordpress.com/2013/09/13/childrenastriggers-in-updatepanel/
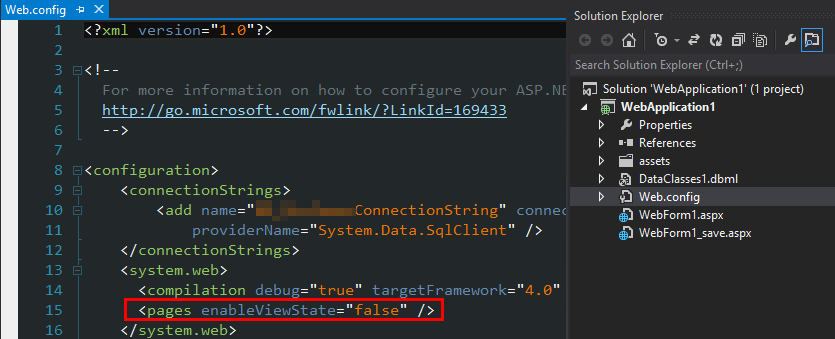